SQL DELETE Statement - The Complete Guide
The SQL DELETE statement allows you to delete records from a table. I'll explain more about the SQL DELETE statement in this article.
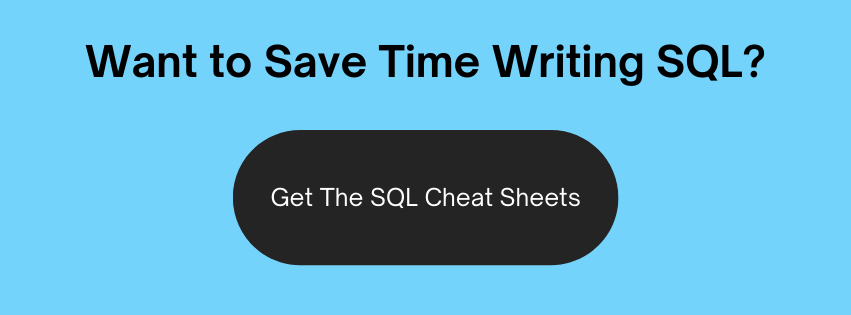
What Is The SQL DELETE Statement?
The SQL DELETE statement, or delete query, is a statement you can run to delete records from a table.
A WHERE clause is used to specify the criteria, and any rows matching these criteria will be deleted. You can use it to delete a single record, multiple records, or all records in a table.
How Can I Write a DELETE Query in SQL?
The DELETE query in SQL has some pretty simple syntax:
1DELETE FROM [ table | (subquery) ] [alias]
2[WHERE condition];
Let me explain this a bit further.
You start with DELETE FROM. There is no need to specify the columns here - we're working on deleting rows so the columns don't matter.
Then, you specify either a table or a subquery. The table here can be either a table, a view, or a materialized view.
Then, you can give the table an alias, to help when writing your WHERE condition.
After this, you can write WHERE then a condition. The condition allows you to specify which rows to delete. If you only want to delete some of the rows in a table, you'll need the WHERE condition. If you want to delete all rows in a table, don't use a WHERE condition.
Examples of an SQL DELETE Query
Let's see some examples. First, let's see some sample data that we can run our DELETE SQL statements on.
1SELECT student_id, first_name, last_name
2FROM student;
STUDENT_ID | FIRST_NAME | LAST_NAME |
---|---|---|
1 | John | Smith |
2 | Susan | Johnson |
3 | Tom | Capper |
4 | Mark | Holloway |
5 | Steven | Webber |
6 | Julie | Armstrong |
7 | Michelle | Randall |
8 | Andrew | Cooper |
9 | Robert | Pickering |
10 | Tanya | Hall |
11 | Jarrad | Winston |
12 | Mary | Taylor |
100 | Mark | Anderson |
102 | John | Rogers |
Example 1 - Simple Delete
This is an example of deleting a single record. Related: How to Delete a Row in SQL.
1DELETE FROM student
2WHERE student_id = 1;
The record that has the student_id of 1 is deleted. All other rows remain in the table.
Example 2 - Using Two Conditions
This example uses two conditions in the WHERE clause.
1DELETE FROM student
2WHERE enrolment_date > '10-JAN-2015'
3AND fees_paid > 400;
11 row(s) deleted.
As you can see, 1 row has been deleted because 1 row matched the WHERE condition.
Can You Delete From Multiple Tables?
Yes, you can in MySQL, but not in other databases.
MySQL Delete From Multiple Tables
To delete records from multiple tables in MySQL, you mention both tables in your Delete statement.
The syntax looks like this:
1DELETE table1, table2
2FROM table1
3INNER JOIN table2 ON table1.field = table2.field
4WHERE condition;
This will delete records from both tables that match your condition.
Let's see an example.
We have two tables, person and company, which contain a few related records.
1CREATE TABLE person (
2 firstname VARCHAR(100),
3 company_id INT
4);
5
6CREATE TABLE company (
7id INT,
8company_name VARCHAR(100)
9);
10
11INSERT INTO person (firstname, company_id) VALUES
12('John', 1), ('Sarah', 2), ('Mark', 1), ('Tania', 3);
13
14INSERT INTO company (id, company_name) VALUES
15(1, 'something'), (2, 'another co'), (3, 'west'), (4, 'northern');
Here's what the data looks like.
Person table:
firstname | company_id |
---|---|
John | 1 |
Sarah | 2 |
Mark | 1 |
Tania | 3 |
Company table:
company_id | company_name |
---|---|
1 | something |
2 | another co |
3 | west |
4 | northern |
You can delete all company and person records that match a specific company using this statement.
1DELETE person, company
2FROM person
3INNER JOIN company ON person.company_id = company.id
4WHERE company.id = 1;
The records are matched between the two tables, and filtered to where company_id = 1;
After this statement is run, these are the results of the tables.
Person table:
firstname | company_id |
---|---|
Sarah | 2 |
Tania | 3 |
Company table:
company_id | company_name |
---|---|
2 | another co |
3 | west |
4 | northern |
Notice how the company ID of 1 is removed, and all person records with a company of 1 have been removed.
Delete from Multiple Tables in Other Databases
In non-MySQL databases, this statement won't work. If you want to delete from multiple tables, you need to write multiple DELETE statements.
1DELETE from student
2WHERE student_id = 1;
3
4DELETE from student_subject
5WHERE student_id = 1;
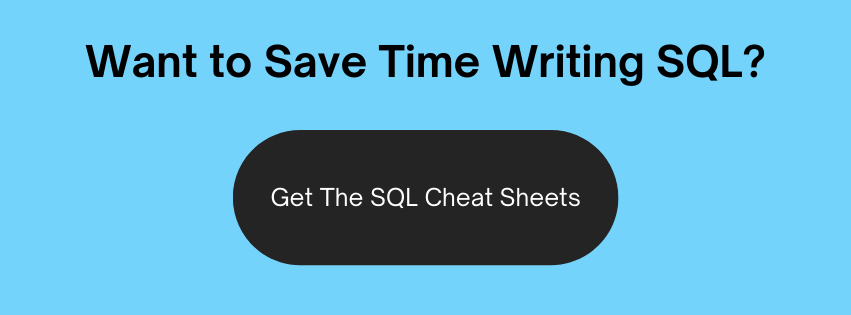
Can You Delete Data Based On Data In Another Table?
Yes, you can.
Let's explain what I mean.
Say, for example, you had your student table. And let's say you wanted to delete all student records that were not in any subjects. This data, though, is in the student_subject table, which links students to subjects.
But, we can't specify more than one table in the SQL DELETE statement, right?
Actually, you can. You just can't delete from more than one table at a time.
You can do that using a join or using a subquery. The method you use may depend on the vendor:
- Oracle: Subquery only, does not support join
- SQL Server: Subquery or join
- MySQL: Subquery or join
- PostgreSQL: Subquery or join
SQL Delete With Subquery
Let's see how we can do this.
1DELETE FROM student
2WHERE student_id NOT IN (
3 SELECT student_subject.student_id
4 FROM student_subject
5);
This query first looks up student_id values in the student_subject table. Then, it deletes records from the student table where the student_id is not in that list.
The method of using a subquery to limit values works in a similar way to the SELECT query. You can use IN, EXISTS, and NOT EXISTS in the same way (read more about these operators here).
SQL Delete With Join
To delete from one table based on data in another table, you can delete using a join. This will work in MySQL, PostgreSQL, and SQL Server.
You can join two tables together in the same way as you would with a SELECT statement, but you just need to specify the name of the table to delete from:
1DELETE student
2FROM student
3INNER JOIN student_subject ON student.student_id = student_subject.student_id
4WHERE student_subject.subject_id = 1;
This will delete all student records that have a student_subject record with a subject_id of 1.
You can also use table aliases to make the query easier to read and write:
1DELETE student
2FROM student st
3INNER JOIN student_subject ss ON st.student_id = ss.student_id
4WHERE ss.subject_id = 1;
This will give you the same result.
Can I See What I'm Deleting Before It Is Deleted?
Yes, you can.
If you want to be sure you're deleting the right records, you can check what you delete before you actually delete the records.
To do this, simply copy the DELETE statement and run it as a SELECT statement first - with the same WHERE clause and everything.
As an example, if we wanted to delete the students that were not enrolled in a subject (just like the previous example), we can check we're deleting the right records first.
The SELECT query is here:
1SELECT * FROM student
2WHERE student_id NOT IN (
3 SELECT student_subject.student_id
4 FROM student_subject
5);
And, the Oracle DELETE query to do that is here:
1DELETE FROM student
2WHERE student_id NOT IN (
3 SELECT student_subject.student_id
4 FROM student_subject
5);
As you can see, I just replaced the DELETE with a SELECT *.
My SQL DELETE Statement Is Too Slow!
Are you trying to delete data from a table but it's too slow?
Well, there are a few things you can do.
First, if you're deleting all of the data in the table, it may be better to use the TRUNCATE TABLE statement. It's faster (because it doesn't write to a log and allow for rollbacks), but you can't undo it, so you need to be sure. You also can't specify a WHERE condition with a TRUNCATE TABLE statement.
If you can't use TRUNCATE, you can try to filter your data differently or use indexes on your table.
Another method is actually creating a new table with the data you want to keep. You can do this by :
- Write a CREATE TABLE AS SELECT method and then specifying the WHERE clause for the records you want to keep
- Delete the original table
- Rename the new table to the old table
Summary of Differences Between Vendors
There are some differences between the way the DELETE statement works with each vendor:
| Criteria | Oracle | SQL Server | MySQL | PostgreSQL | | --- | --- | --- | --- | | Basic DELETE | Follows standard | Follows standard | Follows standard | Follows standard | | Delete with subquery | Allowed | Allowed | Allowed | Allowed | | Delete with join | Not allowed | Allowed | Allowed | Allowed |
Conclusion
So, that's how you run an Oracle DELETE SQL statement. You can delete a single row, multiple rows, or the entire table, using WHERE conditions which can involve subqueries.
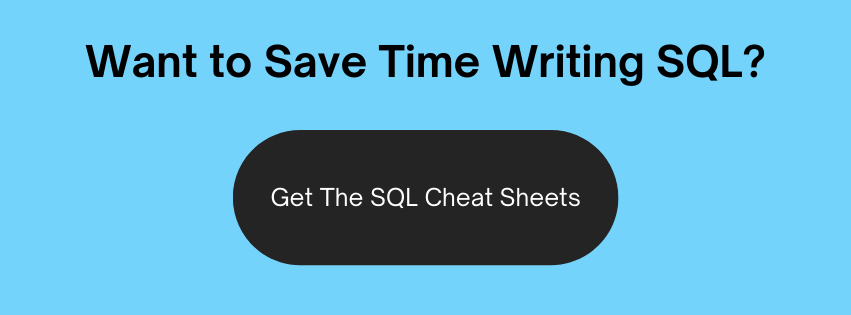